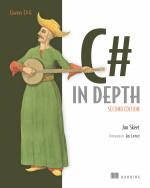
Static vs. Dynamic Typing: A language has static typing if the type of a variable must be known before run time. Compiled languages are statically-typed, whereas dynamic languages are dynamically-typed. For instance, Pascal, C++, and Scala are statically-typed while JavaScript, Ruby, Erlang, and PHP are dynamically-typed. The main advantages of static typing are: avoidance of runtime errors, memory optimization, and IDE features like auto-completion. Dynamically-typed languages let you do things like metaprogramming and duck-typing, which helps with code reuse. Explicit vs. Implicit Typing: Static languages can be either explicitly or implicitly typed. If a language is explicitly-typed, you must declare a variable with a type, such as:
var int = 1;If a language is implicitly-typed, you can declare variables like so:
var = 1;The compiler can figure out what the type is from the context. I'm not sure if there is any advantage to a language being explicitly-typed. Implicit typing improve readability when you have complicated types.
Type-Safe vs. Unsafe Typing: A language is type-unsafe if you can convert completely unrelated types. C and C++ allow you to do this, and there are some odd situations when it might be useful. Type-safe languages prevent you from referencing out-of-bound array locations, converting classes to dates, or adding strings and integers, though the latter could be useful for a language geared for beginners like Visual Basic. The difference between type-safety and type-unsafety is largely a matter of the conversions that a compiler or interpreter knows how to do. If it knows how to turn a string into an integer, and it knows to try this when doing operations with integers and strings, then there should be no issue with adding "5" and 3. However, there is nothing obvious about such conversions. It makes just as much sense to a compiler to add the byte values of the two variables, where you might end up with a value like 119.
Strong vs. Weak Typing: Strong and Weak typing pretty much only refer to collection types. Object arrays in C# are weakly-typed, but other array types are strongly-typed. So, while you could add all kinds of different types to an object array, you could not do this if you first assigned a string array to an object array. This is because the reference is still a string array. I don't know how often anyone would do this, but it suggests that the language of 'strong typing' should be avoided except in rare situations.
It's interesting to note that over time C# has become more and more dynamic, with C# 4 even having a dynamic data type. We now have implicit typing to support data-centric operations through LINQ. As I discussed in a previous post, dynamic typing allows you to mold your data types to those stored in databases without necessarily knowing much about what you're going to get from the database. It's interesting that the TIOBE report categorizes languages according to whether they are static or dynamic. This line is becoming increasingly blurred, just as language paradigms are becoming less rigid as OO languages like Java and C# incorporate elements from functional programming.
Incorporating dynamic typing in compiled languages is part of a trend in which languages are becoming more complex, but also more intuitive and readable. For example, why should you have to know what type a variable will take at code-time, especially when communicating with web services or databases? Though it's easy to just start using LINQ, for example, it's important to understand the problems these developments are meant to solve and the sources of their solutions in order to truly understand them.
No comments:
Post a Comment