
Erlang's simple model is based around processes that pass messages to each other, crash, and respawn very quickly. After compiling a module, you spawn a process using:
handle = spawn(module, function, parameters).The process is defined as a function that shreds out the parameters with a series of case statements:
function -> receive {parameter1} -> %do something {parameter2} -> %do something else Unexpected -> %handle exceptions end.You can pass messages to a spawned process using:
handle ! parameters.This architecture allows you to quickly create, monitor, message, and respawn processes whenever they fail. Because Erlang is a functional language, there are no variables or any other shared resources that can form a bottleneck between processes liked global variables. Processes communicate using messages, and these can be processed asynchronously or independent of any other processes.
Erlang's concurrency model is similar to Service Oriented Architecture, except that concurrency is built into the system at the lowest level, not added on top of an Object Oriented framework and requiring several other technologies. Note also that concurrency is very different from parallelism. If you need to crunch a lot of numbers, you'd need to use a parallel processing system, not a concurrency model.
Erlang is best for soft real-time, distributed, and highly-available applications that could be composed of message-handling systems, like Facebook Chat or low-level telecommunications software. (These are very specific applications that I tend not to think about very often, so I don't have a mock-up like I have tried to have in other posts.)
For these reasons, Erlang is not a cure-all for performance-related problems, though it is important to note that there are limits to any concurrent or parallel approach. Amdahl's law states that even with 95% parallelism, performance benefits will quickly plateau as the number of processors increase. E.g., if your program takes 20 seconds to run, and 1 second of it cannot be parallelized, the least amount of time it could run in is 1 second. No matter how many processors you add, you cannot break that 1 second barrier without further parallelizations.
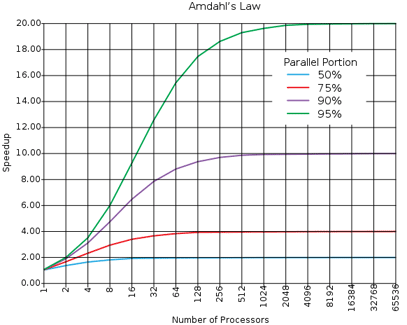
As a side note, I had not heard of Amdahl's law before researching Erlang. Interestingly, I also just learned of IBM's SyNAPSE project, which has the grand aim of functionally replicating the human brain. One argument for a new hardware architecture based on neural networks is that, though the firing of individual neurons is slow, the massively parallel processing power of a brain more than makes up for the performance of any individual part. I'm not sure how this argument stacks up against Amdahl's.
I'll be looking for opportunities to write me some Erlang, but--given my own limits to processing in parallel--this may not be any time soon.
Links:
-Erlang home page
-Eugene Letuchy's notes on designing Facebook Chat
-Learn You Some Erlang, a free online book
Zach,
ReplyDeleteOut of curiosity, can you give some examples of how you see Erlang being used in a business setting? Admittedly, I have zero exposure to it, and haven't done any real multi-threaded or parallel processing type of development work, but I have a system at work that might benefit from something along those lines (specifically, it's a CPU intensive iterative financial calculation over potentially large data sets).
Thanks!
Hi Scout,
ReplyDeleteAnything like that wouldn't really be the best application for Erlang. It's a portmanteau of Ericsson Language and was designed for high availability telecommunications applications. According to Bruce Tate, some Erlang programs have run for years but they can also be hot-swapped out on the fly. Anything that requires a lot of number crunching (like weather analysis) would require a cluster and would probably be best served by C++. If the data sets are databases, then you'd have to rely on the multi-threading built into whatever RDBMS you have. Some RDBMS's now have OO languages built into them to do calculations, which databases aren't so great at. For instance, Oracle has Java; SQL server has the CLR. But I'm not all that knowledgeable about the performance of such applications. Cheers!
Zach
I should add that I was actually a little disappointed in learning what Erlang was good for. There's been a lot of buzz about it, but I can't think of many better uses for it than Facebook chat.
ReplyDeleteZach
OK, that's helpful, thanks.
ReplyDeleteOur current solution is to use a CLR procedure to call a web service, using XML for the data transmission between the two pieces. The problem we run into is that we hit a limit on how many sets we can do the calculation for before the service chokes.
The use of Erlang in telecomm stuff makes sense based on the hot-swappable capability. I wonder if there's anything military-wise that it is used in as well. The ability to swap out components quickly and easily is usually a main requirement for stuff like shipboard systems for the Navy.
Thanks for the review, Zach, much appreciated.
Yeah, I can see how the WS would be a bottleneck. I would try to migrate away from that if possible! If you need realtime response, that may be your only option, but you could look into SS service broker to process the data asynchronously.
ReplyDeleteZach